Years ago when administering a school network there was a requirement for teachers to access student home directories (redirected folders e.g documents, pictures, videos, music ).
In theory this was no problem as teachers had full NTFS permissions over student home folders, however in practice this didn’t work because:
- Teachers didn’t know the UNC paths to student home directories
- Each year group had its own sub folder in this case named after the year the year group left, which teachers didn’t know.
- Due to the folder redirection setup folders didn’t list the same way as the UNC path e.g in file explorer if your home folders were stored under \\server\homefolders\students\2017\ the sub folders within that for each user would display only as “Documents” rather than the username as they were actually named and was used in the UNC path. Very confusing, especially if you are searching through a list of seemingly the same named folders!
So what we need is an easy way for teachers to be able to open a students home directory using a piece of easily available information they did know, a username.
The solution, a VB script.
It needs to be able to take a username input from a teacher via a GUI, look it up in AD and if it exists return the path to the user home folder and open it. So lets see how I did it…
First, we need to start the script and either ask the user for a username via a GUI prompt OR if a username has been specified via the command line then set that as the username.
Set objShell = CreateObject("Wscript.Shell")
On Error Resume Next
' Set the username
If WScript.Arguments.Count = 0 Then
sUser = InputBox("Enter the username", "AD Home Directory Query")
Else
sUser = WScript.Arguments(0)
End If
This is what the user sees after the above code runs.
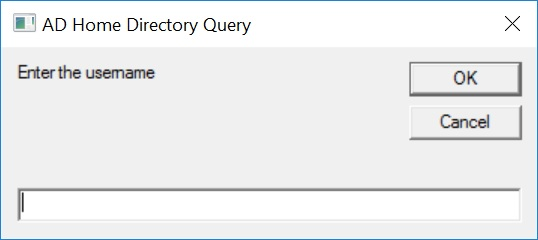
So now we have the username we need to know which domain to search in. If the user has specified a domain above as part of the username e.g DOMAIN\User then we use that, else we grab the current domain used by the machine running the script.
' Check if domain name has been specified
If InStr(sUser, "\") > 0 Then
sDomain = Mid(sUser, 1, InStr(sUser, "\") - 1)
sUser = Mid(sUser, InStr(sUser, "\") + 1)
Else
' If not then use the current domain
sDomain = CreateObject("WScript.Network").UserDomain
End If
Once we have the username and domain name we are ready to query AD.
'Query AD
Set sQuery = GetObject("WinNT://" & sDomain & "/" & sUser & ",user")
Now we need to do some error checking to make sure that firstly the user actually exists and secondly the user has a homefolder set.
' If username does not exist
If Not IsObject(sQuery) Then
MsgBox "Username " & sUser & " could not be found on the " & sdomain & " domain"
Err.Clear
WScript.Quit
End If
' If home directory does not exist for user
If Len(sQuery.HomeDirectory) = 0 Then
MsgBox "No home directory for user " & sQuery.FullName
Err.Clear
WScript.Quit
End If
This is what the user sees if they get errors.
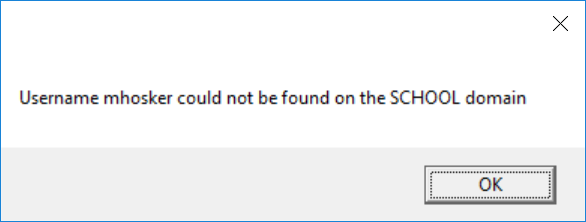
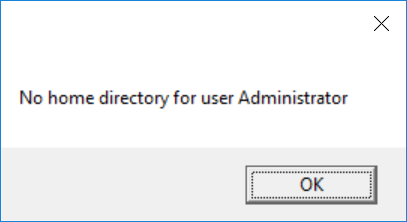
Then if all checks out all that’s left to do is display to the user both the user full name and path to home directory before opening the path in explorer and ending the script.
' If everything else above checks out then we display the username and home directory path
MsgBox (sQuery.FullName & vbCrLf & vbCrLf & sQuery.HomeDirectory)
' Then we open the home directory path in explorer.exe
strPath = "explorer.exe /e," & sQuery.HomeDirectory
objShell.Run sQuery.HomeDirectory
' Finally clear the query object and quit the script
Set sQuery = Nothing
WScript.Quit
So all together you should have:
Set objShell = CreateObject("Wscript.Shell")
On Error Resume Next
' Set the username
If WScript.Arguments.Count = 0 Then
sUser = InputBox("Enter the username", "AD Home Directory Query")
Else
sUser = WScript.Arguments(0)
End If
' Check if domain name has been specified
If InStr(sUser, "\") > 0 Then
sDomain = Mid(sUser, 1, InStr(sUser, "\") - 1)
sUser = Mid(sUser, InStr(sUser, "\") + 1)
Else
' If not then use the current domain
sDomain = CreateObject("WScript.Network").UserDomain
End If
'Query AD
Set sQuery = GetObject("WinNT://" & sDomain & "/" & sUser & ",user")
' If username does not exist
If Not IsObject(sQuery) Then
MsgBox "Username " & sUser & " could not be found on the " & sdomain & " domain"
Err.Clear
WScript.Quit
End If
' If home directory does not exist for user
If Len(sQuery.HomeDirectory) = 0 Then
MsgBox "No home directory for user " & sQuery.FullName
Err.Clear
WScript.Quit
End If
' If everything else above checks out then we display the username and home directory path
MsgBox (sQuery.FullName & vbCrLf & vbCrLf & sQuery.HomeDirectory)
' Then we open the home directory path in explorer.exe
strPath = "explorer.exe /e," & sQuery.HomeDirectory
objShell.Run sQuery.HomeDirectory
' Finally clear the query object and quit the script
Set sQuery = Nothing
WScript.Quit
All that’s left is to find a way to deploy it. In my case I put the script in a shared staff area and copied a shortcut to the script with a custom icon to staff desktops on login.
And that’s it, teachers can now easily access students documents via a nice GUI.